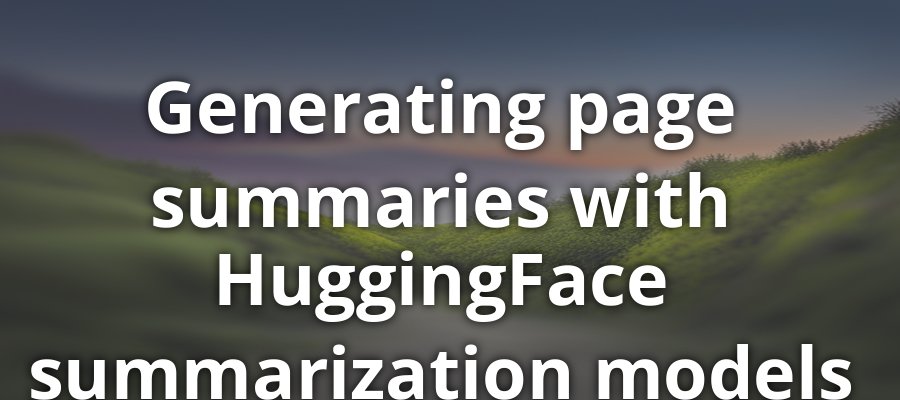
Introduction to Summarization with HuggingFace
In the realm of natural language processing (NLP), the ability to distill extensive texts into concise summaries has become increasingly valuable. This capability not only enhances user experience by providing quick insights but also aids in the efficient management and comprehension of large volumes of information. HuggingFace, a leading innovator in the field of artificial intelligence (AI), offers a suite of models specifically designed for the task of summarization. This section delves into the evolution of summarization models and outlines the objectives behind enhancing page summaries using HuggingFace's technologies.
1.1 The Evolution of Summarization Models
Summarization models have undergone significant transformation, evolving from simple extractive techniques to sophisticated abstractive methods. Initially, summarization was primarily extractive, focusing on identifying and compiling key sentences from a text to form a summary. However, this approach often resulted in summaries that were disjointed or lacked coherence.
The advent of deep learning and transformer-based architectures marked a pivotal shift in summarization technology. Models such as BERT (Bidirectional Encoder Representations from Transformers) and GPT (Generative Pre-trained Transformer) laid the groundwork for more advanced summarization capabilities. HuggingFace's Transformers library, in particular, has been at the forefront of this evolution, providing access to a wide range of pre-trained models optimized for summarization tasks.
Abstractive summarization models, which generate summaries by understanding and rephrasing the content, have become increasingly popular. These models, capable of producing more natural and cohesive summaries, represent the current state-of-the-art in summarization technology. HuggingFace's implementation of models like BART (Bidirectional and Auto-Regressive Transformers) and T5 (Text-to-Text Transfer Transformer) exemplifies the cutting-edge capabilities available today.
1.2 Objective: Enhancing Page Summaries
The primary objective of leveraging HuggingFace summarization models is to enhance the quality and utility of page summaries. In the context of technical documentation, blogs, or informational websites, concise and informative summaries can significantly improve the user's ability to quickly find relevant information. This is particularly important in an era where information overload is a common challenge.
By integrating HuggingFace's summarization models, developers and content creators can automate the generation of accurate and coherent summaries for each page of their site. This not only saves time but also ensures consistency across the entire corpus of content. Furthermore, the flexibility of HuggingFace's API allows for customization and fine-tuning of the summarization process, enabling the creation of summaries that are tailored to the specific needs and preferences of the target audience.
In summary, the evolution of summarization models, spearheaded by advancements in AI and deep learning, has paved the way for more effective and efficient content summarization. HuggingFace's suite of models represents the forefront of this technology, offering powerful tools for enhancing page summaries and improving information accessibility.
Implementing HuggingFace Summarization Models
In this section, we delve into the practical aspects of leveraging HuggingFace's powerful summarization models to generate concise summaries of textual content. The focus is on guiding you through the setup process, selecting the most suitable model for your specific needs, and finally, integrating these models into your application to automate the summarization process. This journey is designed to be accessible for developers and technical professionals aiming to enhance their applications with state-of-the-art summarization capabilities.
Setting Up the Environment
Before diving into the specifics of model selection and integration, it's crucial to establish a robust development environment. This setup involves installing the necessary libraries and dependencies, primarily the Transformers library by HuggingFace, which houses a wide array of pre-trained models including those for summarization.
pip install transformers
This command installs the Transformers library, providing access to the summarization models. It's also advisable to ensure that your Python environment is up-to-date and compatible with the library requirements. For those working within a containerized or virtual environment, ensure that it's properly configured to isolate and manage the dependencies effectively.
Selecting the Right Model for Your Needs
HuggingFace offers several models optimized for summarization tasks, each with its unique characteristics and performance metrics. The choice of model depends on various factors including the desired balance between speed and accuracy, the nature of the input text, and computational resource constraints.
For instance, the facebook/bart-large-cnn
model is renowned for its balance between performance and efficiency, making it a popular choice for summarization tasks:
from transformers import pipeline
summarizer = pipeline("summarization", model="facebook/bart-large-cnn")
This code snippet initializes the summarization pipeline using the facebook/bart-large-cnn
model. It's a starting point for experimenting with summarization capabilities, allowing for adjustments and fine-tuning based on specific requirements.
Integrating Summarization into Your Application
With the environment set up and the model selected, the next step is to integrate the summarization functionality into your application. This process involves feeding text to the model and processing the output to achieve the desired summary.
def generate_summary(text):
summary = summarizer(text, max_length=130, min_length=30, do_sample=False)
return summary[0]['summary_text']
This function, generate_summary
, takes a piece of text as input and uses the previously initialized summarizer
pipeline to generate a summary. The parameters max_length
and min_length
control the length of the summary, while do_sample
determines whether sampling is used to generate the summary.
Integrating this function into your application allows for dynamic summarization of content, enhancing the user experience by providing concise and relevant summaries of extensive textual information.
In conclusion, implementing HuggingFace summarization models involves setting up the development environment, selecting the appropriate model based on your needs, and integrating the summarization capability into your application. By following these steps, developers can harness the power of advanced NLP models to enhance their applications with automated text summarization features.
Optimizing Summarization Performance
In this section, we delve into the critical aspects of optimizing the performance of summarization models, particularly those provided by HuggingFace. The focus is on two main areas: evaluating model accuracy and efficiency, and handling large texts and documents. By addressing these areas, we aim to enhance the effectiveness of generating page summaries using HuggingFace summarization models.
3.1 Evaluating Model Accuracy and Efficiency
Evaluating the accuracy and efficiency of a summarization model is paramount to ensuring its effectiveness in real-world applications. Accuracy refers to the model's ability to generate summaries that closely reflect the content and intent of the original text, while efficiency pertains to the model's speed and resource consumption during the summarization process.
Accuracy Metrics
To assess accuracy, several metrics can be employed, including ROUGE (Recall-Oriented Understudy for Gisting Evaluation) and BLEU (Bilingual Evaluation Understudy). ROUGE measures the overlap of n-grams between the generated summary and a set of reference summaries, providing insights into the precision, recall, and F1-score of the model. BLEU, on the other hand, evaluates the quality of machine-generated text by calculating the precision of n-grams in the generated text against reference texts.
from rouge import Rouge
from nltk.translate.bleu_score import sentence_bleu
# Example code to calculate ROUGE score
rouge = Rouge()
scores = rouge.get_scores(generated_summary, reference_summary)
# Example code to calculate BLEU score
score = sentence_bleu([reference_summary.split()], generated_summary.split())
Efficiency Considerations
Efficiency can be gauged through metrics such as inference time and memory usage. Optimizing these aspects involves techniques like model quantization, which reduces the precision of the model's parameters to accelerate inference while minimally impacting accuracy. Another approach is pruning, which eliminates redundant or non-contributory parameters from the model.
3.2 Handling Large Texts and Documents
Summarizing large texts and documents presents unique challenges, primarily due to the input length restrictions of most transformer-based models. A common strategy to address this involves segmenting the text into smaller chunks that fit the model's maximum input size, summarizing each chunk independently, and then concatenating the summaries.
Chunking Strategy
The chunking strategy involves dividing the input text into segments that do not exceed the model's maximum input length. This approach ensures that important context is not lost and that the summarization model can process each segment effectively.
def chunk_text(text, max_length):
chunks = []
current_chunk = []
current_length = 0
for sentence in nltk.sent_tokenize(text):
sentence_length = len(sentence.split())
if current_length + sentence_length > max_length:
chunks.append(' '.join(current_chunk))
current_chunk = [sentence]
current_length = sentence_length
else:
current_chunk.append(sentence)
current_length += sentence_length
chunks.append(' '.join(current_chunk))
return chunks
Summarization and Reassembly
After chunking, each segment is fed into the summarization model to generate a summary. These individual summaries are then concatenated to form the final comprehensive summary of the entire text.
def summarize_chunks(chunks, summarizer):
summaries = [summarizer(chunk) for chunk in chunks]
final_summary = ' '.join(summaries)
return final_summary
By evaluating model accuracy and efficiency and implementing effective strategies for handling large texts, we can significantly enhance the performance of HuggingFace summarization models. These optimizations ensure that the generated summaries are both accurate and generated within acceptable time frames, even for extensive documents.
Advanced Techniques and Considerations
In this section, we delve into the nuanced aspects of summarization technology, focusing on the comparison between abstractive and extractive summarization methods, and exploring the horizon of future trends in this rapidly evolving field. Our aim is to equip readers with a deeper understanding of the advanced techniques that drive the development of summarization models and to provide insights into what the future holds for this technology.
Exploring Abstractive vs. Extractive Summarization
Abstractive Summarization: A Creative Approach
Abstractive summarization models generate summaries by understanding the main ideas in the text and expressing them in new words. Unlike extractive summarization, which simply selects and concatenates portions of the source text, abstractive models have the ability to paraphrase and rephrase, often leading to more coherent and fluent summaries. This approach relies heavily on advanced natural language processing (NLP) techniques and deep learning models, such as sequence-to-sequence architectures and attention mechanisms.
One of the most notable advancements in abstractive summarization is the use of transformer-based models, such as BERT and GPT, which have significantly improved the quality of generated summaries. These models are trained on vast amounts of text data, enabling them to generate summaries that are not only accurate but also maintain the stylistic and tonal nuances of the original text.
from transformers import pipeline
summarizer = pipeline("summarization", model="t5-base")
text = """Your long text document here."""
summary = summarizer(text, max_length=50, min_length=25, do_sample=False)
print(summary[0]['summary_text'])
Extractive Summarization: The Art of Selection
Extractive summarization models identify and extract key sentences or phrases from the source text to create a summary. This method is grounded in the principle that the most important information can be directly lifted from the text without the need for rephrasing. Techniques such as sentence scoring based on word frequency, position, and similarity to other sentences in the text are commonly used to determine the relevance of each sentence.
While extractive summarization is generally faster and easier to implement than abstractive summarization, it may result in less natural-sounding summaries, as the extracted sentences are taken directly from the source text without modification.
from sumy.parsers.plaintext import PlaintextParser
from sumy.nlp.tokenizers import Tokenizer
from sumy.summarizers.lsa import LsaSummarizer
text = """Your long text document here."""
parser = PlaintextParser.from_string(text, Tokenizer("english"))
summarizer = LsaSummarizer()
summary = summarizer(parser.document, 3) # Summarize to 3 sentences
for sentence in summary:
print(sentence)
Future Trends in Summarization Technology
The future of summarization technology is poised for transformative advancements, driven by the continuous evolution of machine learning algorithms and the increasing availability of diverse datasets. One emerging trend is the integration of multimodal inputs, such as combining text with images or videos to generate more comprehensive and engaging summaries. This approach could revolutionize content consumption, making it more accessible and informative.
Another area of focus is the development of more personalized summarization models. By leveraging user data and preferences, these models could tailor summaries to the individual needs and interests of each user, enhancing the relevance and utility of the summarized content.
Furthermore, as concerns about bias and fairness in AI grow, future summarization models will likely incorporate mechanisms to detect and mitigate bias in generated summaries. This will be crucial in ensuring that summarization technology serves a diverse global audience in an equitable manner.
In conclusion, the field of summarization is on the cusp of significant breakthroughs, with advanced techniques and considerations shaping the future of how we process and consume information. As we continue to explore the depths of abstractive and extractive summarization, and as we anticipate the exciting developments on the horizon, it is clear that summarization technology will play a pivotal role in the evolution of information dissemination and consumption.
Conclusion
In this article, we have embarked on a comprehensive journey through the landscape of text summarization, with a particular focus on leveraging HuggingFace's powerful summarization models. Our exploration spanned from the foundational concepts and evolution of summarization models to the practical implementation and optimization of these models for generating concise, informative summaries. As we conclude, let's encapsulate the key insights gained and look ahead to the future of summarization technology.
Summarizing Key Takeaways
The realm of text summarization has witnessed significant advancements, primarily driven by the development of sophisticated models like those offered by HuggingFace. We began by understanding the essence of summarization and its critical role in distilling vast amounts of information into digestible, actionable insights. The evolution of summarization models was charted, highlighting the transition from extractive to abstractive techniques, each with its unique approach to condensing text.
Our discussion then shifted to the practical aspects of implementing these models. We detailed the process of setting up the environment, selecting the appropriate model for specific needs, and integrating summarization capabilities into applications. This hands-on guide was designed to equip readers with the knowledge to harness the power of HuggingFace's models effectively.
Optimizing the performance of summarization models was another focal point. We delved into evaluating model accuracy and efficiency, addressing challenges such as handling large texts and documents. This section underscored the importance of fine-tuning and adapting models to meet the demands of diverse summarization tasks.
Lastly, we explored advanced techniques and considerations, including the comparison between abstractive and extractive summarization and the anticipation of future trends in the field. This discussion aimed to broaden the horizon of possibilities and encourage innovation in the application of summarization technology.
Next Steps in Summarization Technology
Looking forward, the field of text summarization is poised for further breakthroughs. The continuous evolution of machine learning algorithms and the increasing computational power at our disposal are set to unlock new capabilities and applications. We can anticipate more nuanced and context-aware summarization models that better understand the subtleties of language and narrative flow.
Moreover, the integration of summarization technology into a wider array of platforms and tools will likely become more seamless. This will enable users across various domains to leverage summarization effortlessly, enhancing productivity and information accessibility.
In conclusion, the journey through the landscape of text summarization with HuggingFace models has been enlightening and empowering. As we stand on the brink of new advancements, the potential for innovation in generating and utilizing text summaries is boundless. The key takeaways and insights shared in this article serve as a foundation upon which to build and explore the exciting future of summarization technology.