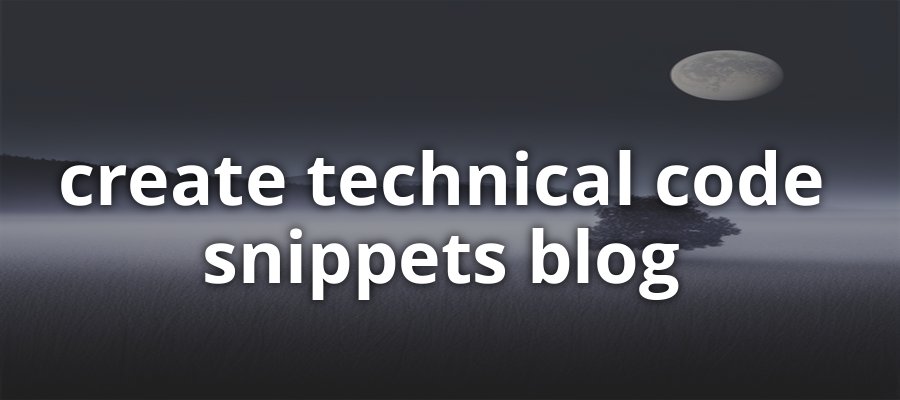
Understanding Code Snippets in Technical Blogs
1.1 The Importance of Code Snippets for Developers
Code snippets serve as critical tools for developers, offering concise examples of syntax and functionality within programming documentation and tutorials. They enable developers to quickly grasp complex concepts by providing tangible, executable pieces of code. This practical approach to learning and problem-solving is essential in a field where theoretical knowledge must be applied to real-world scenarios. Furthermore, code snippets facilitate the transfer of knowledge between experienced and novice programmers, acting as a bridge that accelerates the learning curve.
Incorporating code snippets into technical blogs not only enhances comprehension but also encourages experimentation. Developers can modify and test these snippets, leading to a deeper understanding of the subject matter. Additionally, snippets act as a reference point, allowing developers to revisit and review code that they may need to implement in their projects.
1.2 Best Practices for Writing and Formatting Code Snippets
When creating code snippets for technical blogs, clarity and precision are paramount. The following best practices should be adhered to:
-
Simplicity: Strive for minimalism by including only the necessary code required to demonstrate a concept or solve a problem. Extraneous code can distract and confuse the reader.
-
Context: Provide a brief explanation of what the code does and why it is necessary. Contextual information helps readers understand the relevance and application of the snippet.
-
Syntax Highlighting: Use syntax highlighting to improve readability. Differentiating keywords, variables, and strings through color-coding can significantly reduce cognitive load.
-
Comments: Include comments within the code to explain complex or non-obvious parts of the snippet. Comments should be concise and add value to the reader's understanding.
-
Error Handling: Demonstrate robust coding practices by including error handling within snippets. This not only educates readers on best practices but also ensures that the provided code is more practical for real-world application.
-
Consistency: Maintain a consistent coding style throughout the blog to avoid confusion. This includes consistent naming conventions, indentation, and bracket placement.
-
Testing: Before publishing, test code snippets to ensure they work as intended. This step is crucial to maintain credibility and trust with the audience.
By following these guidelines, technical writers can create code snippets that are not only informative but also practical and user-friendly, thereby enhancing the overall value of their technical blogs.
Creating and Integrating Syntax-Highlighted Snippets
2.1 Selecting the Right Tools for Syntax Highlighting
When preparing to integrate syntax-highlighted code snippets into technical blogs, the selection of appropriate tools is paramount. Syntax highlighting enhances readability and comprehension for developers by visually differentiating elements of the code. Prism.js and Google-Code-Prettify are two widely recognized libraries that serve this purpose. Prism.js is known for its extensibility and support for a wide range of languages, while Google-Code-Prettify is lauded for its ease of use and implementation. Both libraries offer a range of themes to match the aesthetic of the host blog. It is essential to consider factors such as supported languages, customization options, and performance implications when choosing a syntax highlighting tool.
2.2 Step-by-Step Guide to Embedding Code Snippets
Embedding code snippets with syntax highlighting into a blog post involves several key steps. First, ensure that the chosen syntax highlighting library is compatible with the blog platform. For instance, if using Prism.js, include the necessary CSS and JavaScript files from the Prism.js CDN. Next, within the blog post's HTML, use the <pre>
and <code>
tags to wrap the code snippet. Assign appropriate classes to these tags based on the language of the code and the desired syntax highlighting theme.
Here is an example of embedding a JavaScript snippet using Prism.js:
<link href="https://cdn.jsdelivr.net/npm/prismjs@1.23.0/themes/prism-okaidia.css" rel="stylesheet" />
<script src="https://cdn.jsdelivr.net/npm/prismjs@1.23.0/prism.js"></script>
<pre><code class="language-javascript">
// Sample JavaScript code
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet('World');
</code></pre>
After adding the code snippet, verify its appearance in the blog's preview mode to ensure proper rendering. Adjust the styling as necessary to align with the blog's design. Finally, test the blog post across different browsers and devices to confirm the consistency of the syntax highlighting.
Advanced Techniques for Code Snippets
In the realm of technical blogging, code snippets serve as the bridge between abstract concepts and practical implementation. They are not merely excerpts of code; they are educational tools that, when leveraged with advanced techniques, can significantly enhance the learning experience of the reader. This section delves into sophisticated methods for elevating the utility and effectiveness of code snippets within technical content.
3.1 Dynamic Code Snippets: Enhancing Interactivity
Dynamic code snippets are interactive elements that allow users to modify and execute code within the blog post itself. This interactivity can lead to a deeper understanding of the code's functionality and its potential applications.
To implement dynamic snippets, one must integrate a JavaScript-based engine or framework that supports code execution in a secure sandbox environment. Such environments isolate code execution to prevent any potential security risks. Examples include JSFiddle, CodePen, or custom implementations using web technologies like WebAssembly for languages other than JavaScript.
Consider the following example:
// Dynamic snippet allowing user interaction
function greet(name) {
return `Hello, ${name}!`;
}
// Placeholder for user input
let userName = 'Developer';
// Executable snippet
console.log(greet(userName));
In this snippet, users could be given the option to change the userName
variable and observe different outputs. This not only makes the learning process more engaging but also empowers readers to experiment with the code, leading to better retention of information.
3.2 Optimizing Code Snippets for SEO and Readability
Optimizing code snippets for search engines (SEO) and readability is crucial for reaching a wider audience and ensuring that the content is accessible and understandable.
For SEO, it is important to include relevant keywords within the code comments and explanations. This practice helps search engines understand the context of the code and index it appropriately. Additionally, using schema.org markup for software source code can provide search engines with structured information about the code snippet.
Readability can be enhanced by adhering to coding standards and best practices, such as consistent indentation, meaningful variable names, and concise comments that explain the purpose and functionality of the code. For instance:
# SEO-optimized and readable Python snippet for a Fibonacci sequence
def fibonacci(n):
"""Generate a Fibonacci sequence up to the nth value"""
sequence = [0, 1]
while len(sequence) < n:
sequence.append(sequence[-1] + sequence[-2])
return sequence
# Example usage with a descriptive comment
# Generates the first 10 numbers in the Fibonacci sequence
print(fibonacci(10))
In this Python snippet, the function fibonacci
is clearly documented with a docstring, and the example usage includes a comment that aids comprehension. The inclusion of terms like "Fibonacci sequence" and "generate" within the comments can also assist with SEO.
By applying these advanced techniques, technical bloggers can create code snippets that not only convey information effectively but also engage and educate their readers in a more profound manner.
Language-Specific Snippet Guides
Technical blogs often serve as a critical resource for developers seeking to enhance their skills or solve specific problems. Language-specific code snippets are instrumental in this learning process, providing actionable examples that can be directly applied to real-world scenarios. This section delves into the creation and application of code snippets for two widely-used programming languages: Python and JavaScript.
4.1 Python Snippets for Data Science and Machine Learning
Python has become the lingua franca of data science and machine learning due to its simplicity and the vast ecosystem of libraries and frameworks it supports. When crafting Python snippets for technical blogs, it is essential to focus on clarity and efficiency. The snippets should leverage libraries such as NumPy for numerical operations, pandas for data manipulation, and scikit-learn for machine learning algorithms.
import numpy as np
from sklearn.linear_model import LinearRegression
# Sample data
X = np.array([[1, 1], [1, 2], [2, 2], [2, 3]])
y = np.dot(X, np.array([1, 2])) + 3
# Linear regression model
model = LinearRegression().fit(X, y)
# Predictions
predictions = model.predict(X)
The above snippet demonstrates a simple linear regression model using scikit-learn. It is concise, yet complete, allowing developers to quickly grasp the concept and apply it to their datasets.
4.2 JavaScript Snippets for Web Development
JavaScript is the backbone of web development, enabling interactive and dynamic user interfaces. When presenting JavaScript snippets, it is crucial to address both vanilla JavaScript and popular frameworks or libraries like React or Vue.js. The snippets should illustrate common tasks such as DOM manipulation, event handling, and state management.
document.addEventListener('DOMContentLoaded', (event) => {
const button = document.querySelector('#myButton');
button.addEventListener('click', () => {
alert('Button clicked!');
});
});
This snippet sets up an event listener for the DOMContentLoaded event, ensuring the JavaScript code runs only after the HTML document has been fully loaded. It then adds a click event listener to a button with the ID myButton
, displaying an alert when clicked.
By providing targeted, language-specific code snippets, technical blogs can empower developers to quickly learn and implement new techniques in their respective fields. These snippets serve as both educational tools and practical solutions, fostering a community of continuous learning and development.
Maintaining and Sharing Your Code Snippets
5.1 Version Control and Collaboration with Snippets
Effective management of code snippets is crucial for developers, particularly when collaborating on projects. Version control systems (VCS) such as Git provide a robust framework for tracking changes, branching, and merging code. When storing snippets in a VCS, developers should adhere to best practices such as committing small, atomic changes with clear messages. This facilitates easier code reviews and rollback if necessary.
For collaboration, platforms like GitHub, GitLab, and Bitbucket offer features like pull requests and merge requests. These features enable peer review of code snippets before integration into the main codebase. Additionally, using a README file or a dedicated documentation directory within the repository can help collaborators understand the purpose and usage of various snippets.
To illustrate, consider the following example of a Git commit command with a descriptive message:
git commit -m "Add minimum viable snippet for user authentication"
This command commits a new code snippet to the repository with a message that clearly describes its purpose, aiding in collaboration and future maintenance.
5.2 Leveraging Communities for Feedback and Improvement
Sharing code snippets with developer communities can be a powerful way to receive feedback and foster improvement. Platforms such as Stack Overflow, Reddit, and specialized forums are frequented by experienced developers who can offer insights and suggest enhancements.
When sharing snippets, it is important to provide context and ask specific questions. This approach encourages constructive feedback and detailed responses. For example, posting a snippet along with a question about optimizing its performance is more likely to yield useful advice than simply sharing the code without any accompanying information.
Here is a template for sharing a code snippet on a forum for feedback:
Title: Optimizing Array Sorting Function for Large Datasets
Hello everyone,
I've been working on an array sorting function that needs to handle large datasets efficiently. Below is the snippet I'm currently using:
```javascript
function sortLargeDataset(dataset) {
// Sorting logic here
}
I'm looking for advice on how to improve the performance of this function. Are there any best practices or algorithms that I should consider?
Thank you in advance for your help!
By following these guidelines, developers can create, maintain, and share technical code snippets effectively, ensuring that their work remains organized, accessible, and open to continuous refinement.